While Loop Explained with Syntax and Examples of C programming
In general, statements in a program are executed sequentially. The first statement is executed first, followed by the second, and so on. But in some situations, it is required to execute a block of code over and over again. Loops are used to execute a block of statements a specified number of times until a condition is met.
C supports the following types of loops:
for loop (CLICK HERE)
while loop
do-while loop
WHILE LOOP:
The most basic loop in C is the while loop. It is controlled by a condition and is used to repeat a block of code until the condition is true.
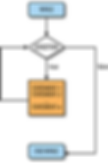
Syntax:
while (condition)
{
statement(s);
}
The loop gets executed when the condition is true and is repeated until the condition becomes false. When the condition becomes false, the program control passes to the line immediately following the loop.
NOTE:
It is not compulsory for a while loop to be executed at all.
When the condition is tested and the result is false at the first time itself then the loop body will be skipped and the first statement after the while loop will be executed.
Examples:
To print the first 20 even numbers
#include<stdio.h>
int main()
{
int n;
n=2;
while( n<=20 )
{
printf("\n%d",n);
n = n+2;
}
return 0;
}